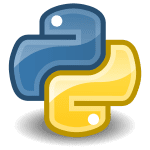
Software tools are a cornerstone of modern Reliability Engineering, enabling reliability practitioners to perform their analysis without getting bogged down in the details of the underlying mathematical processes. There are many software tools available for reliability engineering, some of which are tailored to this application, while others are more general statistical tools which can be adapted to the needs of reliability engineers. One thing these tools have in common is their graphical user interface (GUI). The GUI requires only a basic level of knowledge to operate, but with a few clicks of the correct buttons, the desired task can be achieved with relatively little mental effort. It is the user friendly GUI that draws reliability engineers to select such applications as their tools of choice for performing reliability engineering analyses.
Programming languages such as Python, R, and Matlab may seem daunting to anyone unfamiliar with the specific programming language, or with programming in general. Unfamiliarity with programming can create a barrier to entry into a world of analyses that far exceeds the capabilities of any GUI based software tool. Everything that can be done in a GUI based tool is possible to do in a programming language. This should seem obvious since every GUI based tool is written atop a programming language, but what is not immediately obvious is the limitations of GUI based tools due to their base programming. Programming languages do not suffer from such limitations, as their capabilities are only limited by the programming ability of the user.
What many users may not be aware of is programming libraries, which provide some middle ground between GUI based software and pure code. Libraries offer a collection of functions which accept inputs and give outputs, typically executed using a single line of code. Users unfamiliar with programming do not need to learn much in order to utilize these libraries, particularly if they are well documented with examples and application programming interface (API) documentation. If you have not used programming languages before or are unfamiliar with them, consider giving the examples in this article a try just to see how simple programming can be through the use of libraries. This article is the first in a series that aims to introduce users to one such library in Python called reliability.
But why should I bother, you might ask? Remember that you don’t know what you don’t know. Before dismissing programming as too much trouble, take the time to learn a little about its advantages over GUI based applications. You’ll soon see why programming is more accessible than ever before, and how it can enable you as a reliability engineer to free yourself from the bounds of what your existing software was hardcoded to do. Reliability engineering also has some crossover with data science and machine learning. Programming languages are extremely useful tools for data scientists, and as you’ll see in this series, they can also be of great use to reliability engineers.
Before we get started, let’s look at a few advantages of using Python for reliability engineering when compared to other programming languages and GUI based software tools:
- Free
- Open source (you can see how the code works and modify it if you want)
- Versatile (extremely flexible in what it can be made to do)
- Scriptable (easy to automated analyses if you do them regularly)
- Large online community willing to help
- Extensive collection of libraries
- Rapid development cycle to introduce new features
- Cross domain skillset with applications in data science, machine learning, simulation, etc.
- Easy to learn compared to most other programming languages
- In demand skillset
Getting started with Python
To install Python (if it is not already installed), you’ll need to be working on a computer to which you have administrator rights. There are many tutorials online that will walk you through the installation of Python. The most important thing to understand is that you need Python (the language) and you also need an Interactive Development Environment (IDE). The IDE lets you see the code and execute it much more easily. Without the IDE you’d only be able to interact with Python using the command line, which is no fun. I highly recommend PyCharm as the IDE.
Once you have Python installed, you’ll need to install reliability. The easiest way to do this is to open your terminal / command prompt and type:
pip install reliability
Everything from here will assume you’ve got reliability installed and you are able to execute code.
Getting started with the python reliability library
Let’s start with the most common example that you’d probably use software for; fitting a Weibull distribution to some failure times.
from reliability.Fitters import Fit_Weibull_2P
Fit_Weibull_2P(failures=[5,7,12,15])
In the above code we import the function “Fit_Weibull_2P” from the Fitters module within reliability. We then call the function and pass a list of failure times [5,7,12,15] to the argument “failures”. That’s it! When we hit run, we get the following output:
Results from Fit_Weibull_2P (95% CI):
Analysis method: Maximum Likelihood Estimation (MLE)
Failures / Right censored: 4/0 (0% right censored)
Parameter Point Estimate Standard Error Lower CI Upper CI
Alpha 11.0171 2.11865 7.55747 16.0605
Beta 2.74572 1.11779 1.23632 6.09791
Goodness of fit Value
Log-likelihood -11.0406
AICc 38.0812
BIC 24.8538
AD 2.96746
From this example you can see that all the hard work is done by the reliability library and there is only a basic level of knowledge required to call the function, structure the data in a list, and pass the list to the correct argument within the function.
While most libraries in Python are non-GUI based, the plotting library matplotlib is GUI based so you can interact with the plots to zoom, scroll, save the figure, etc. For many of the functions within reliability, there is a plot produced. Depending on whether you’re using a Jupyter notebook or a regular IDE like PyCharm, your plot may be displayed automatically or it may need to be called. Let’s repeat the example above, but this time use some more data, some of which is right censored.
from reliability.Fitters import Fit_Weibull_2P
import matplotlib.pyplot as plt
failures = [37, 32, 41, 18, 41, 24, 25, 27, 62, 42, 68, 29, 22, 49, 14, 37, 31, 23, 15, 16, 12]
right_censored = [28, 1, 4, 66, 11, 35, 24, 3, 30]
Fit_Weibull_2P(failures = failures,right_censored=right_censored, print_results=False)
plt.show()
The output from this code block is:

The extra thing we needed to do to get the plot was import the plotting library “matplotlib” and call the plot using plt.show(). We also turned off printing the results. A lot of Python functions work using the dot operator so you will often see commands like “from A.B import C” or operations like “A.B()”. You’ll soon get accustom to the syntax of Python if you’re not already.
Let’s view that plot a little differently. If we wanted to see the survival function (often called the “reliability function” R(t)) then we would do the following:
from reliability.Fitters import Fit_Weibull_2P
import matplotlib.pyplot as plt
from reliability.Probability_plotting import plot_points
failures = [37, 32, 41, 18, 41, 24, 25, 27, 62, 42, 68, 29, 22, 49, 14, 37, 31, 23, 15, 16, 12]
right_censored = [28, 1, 4, 66, 11, 35, 24, 3, 30]
results = Fit_Weibull_2P(failures = failures,right_censored=right_censored, print_results=False,show_probability_plot=False)
results.distribution.SF()
plot_points(failures=failures,right_censored=right_censored,func='SF')
plt.show()

The only differences here are that we turned off the probability plot, stored the results from the fitter in a variable called “results”, called the survival function from the distribution object that’s produced with the results, and called a function named plot_points to produce the scatter plot of the failures. At first glance, this might sound complicated but it is really only a few extra steps beyond the previous example.
Working with objects in Python
An object is a data structure that can have certain properties and also contain sub-functions. Within reliability we can create a Weibull Distribution object and quickly do some common tasks using that object.
from reliability.Distributions import Weibull_Distribution
import matplotlib.pyplot as plt
dist = Weibull_Distribution(alpha=5,beta=2)
print("The mean is:", dist.mean)
dist.PDF()
plt.show()
This gives the following output:
The mean is: 4.4311346272637895

In the above code block we created a Weibull Distribution object which we assigned to the variable “dist”. To create the Weibull Distribution we only needed to specify two parameters, alpha (the scale parameter) and beta (the shape parameter). The distribution object automatically calculates a number of properties such as mean, median, mode, variance, skewness, kurtosis, etc. These can be called using the dot operator as shown with “dist.mean”.
The distribution object has a number of internal functions. One of these is the PDF which generates a plot. The PDF function will also return the y-values of the plot, though the above example does not show this. Other important functions within each distribution object include CDF, SF, HF, CHF, quantile, random_samples, and many more. See the API documentation for a full list.
Something more advanced
So far we have seen how Python can be used to replicate some of the functionality you’ve likely seen in GUI based programs. Let’s now take a look at a more complicated example where Python’s scriptable nature makes it a tool of choice for such analyses. In the below example we will generate some random data from a distribution, fit all 12 distributions to the data and take the best one. The plots from fitting everything are turned off for this example. Then we will use that best fitting distribution as our strength distribution. We will repeat the process for another dataset to get our strength distribution. We will then perform a stress-strength interference analysis to determine the probability of failure. The output from the following code block provides a few text outputs and the stress-strength interference plot. Doing this in a GUI based program is certainly possible, but it would be much more time consuming and difficult to get the information to flow between functions without needing to manually transcribe it.
from reliability.Distributions import Gamma_Distribution, Lognormal_Distribution
from reliability.Fitters import Fit_Everything
from reliability.Other_functions import stress_strength
import matplotlib.pyplot as plt
strength_data = Gamma_Distribution(alpha=50, beta=8).random_samples(20, seed=1)
stress_data = Lognormal_Distribution(mu=5,sigma=0.8) .random_samples(30, seed=2)
strength_fit = Fit_Everything(failures = strength_data, show_best_distribution_probability_plot=False,show_probability_plot=False, show_PP_plot=False, show_histogram_plot=False)
stress_fit = Fit_Everything(failures = stress_data, show_best_distribution_probability_plot=False,show_probability_plot=False, show_PP_plot=False, show_histogram_plot=False)
stress_strength(stress = stress_fit.best_distribution, strength=strength_fit.best_distribution)
plt.show()
The above code block gives the following output:
Results from Fit_Everything:
Analysis method: MLE
Failures / Right censored: 20/0 (0% right censored)
Distribution Alpha Beta Gamma Mu Sigma Lambda Log-likelihood AICc BIC AD
Lognormal_2P 5.88324 0.387089 -127.061 258.828 260.114 1.41411
Gamma_2P 57.1622 6.77347 -127.406 259.517 260.803 1.53574
Loglogistic_2P 351.37 4.33532 -127.754 260.213 261.499 1.47015
Weibull_3P 262.946 1.56249 150.803 -126.711 260.921 262.408 1.35448
Exponential_2P 163.008 0.00446074 -128.249 261.203 262.489 2.14135
Gamma_3P 98.6681 2.56781 133.825 -126.787 261.073 262.561 1.32766
Weibull_2P 437.325 2.73022 -128.316 261.337 262.623 1.62373
Lognormal_3P 51.9464 5.71166 0.456422 -126.925 261.35 262.837 1.34482
Loglogistic_3P 227.688 2.85063 116.652 -127.294 262.089 263.576 1.26601
Normal_2P 387.186 153.338 -129.032 262.769 264.055 1.82908
Gumbel_2P 468.019 157.61 -131.46 267.625 268.911 1.95383
Exponential_1P 0.00286052 -139.286 280.794 281.568 5.0292
Results from Fit_Everything:
Analysis method: MLE
Failures / Right censored: 30/0 (0% right censored)
Distribution Alpha Beta Gamma Mu Sigma Lambda Log-likelihood AICc BIC AD
Loglogistic_2P 108.276 2.3906 -176.01 356.464 358.821 0.774241
Lognormal_2P 4.73531 0.760156 -176.398 357.241 359.599 0.83567
Gamma_3P 202.783 0.659162 26.8708 -174.816 356.556 359.836 1.72922
Loglogistic_3P 83.8322 1.82108 21.2611 -175.068 357.059 360.34 0.596265
Exponential_2P 26.8708 0.00748136 -176.86 358.165 360.523 1.24987
Weibull_3P 118.706 0.79152 26.8708 -175.273 357.469 360.749 1.57376
Lognormal_3P 17.6694 4.49486 0.938032 -175.495 357.913 361.193 0.688376
Gamma_2P 100.155 1.6029 -180.597 365.638 367.996 1.59745
Exponential_1P 0.00622908 -182.356 366.854 368.113 2.06219
Weibull_2P 170.586 1.1525 -181.767 367.979 370.337 1.77386
Normal_2P 160.537 177.812 -197.99 400.425 402.783 4.12004
Gumbel_2P 269.578 284.701 -211.034 426.512 428.87 5.70553
Stress - Strength Interference
Stress Distribution: Loglogistic Distribution (α=108.276,β=2.3906)
Strength Distribution: Lognormal Distribution (μ=5.8832,σ=0.3871)
Probability of failure (stress > strength): 8.93989 %

The above example shows how data can flow between functions much more easily than is typically possible using a GUI based tool, and with the addition of a data import to replace the random sampling, this code could form part of an automated process that a manufacturing facility runs every day as part of their quality assurance processes.
Conclusion
The Python library reliability bridges the gap between traditional GUI based programs and pure code. The functions within the library provide reliability engineers with a suite of tools that can perform analysis equivalent to most GUI based software tools. If you have never tried programming or you were not aware that such a tool existed in Python, I highly encourage you to give it a try. It takes some learning at first, but the scriptable nature of programming languages enables you to free yourself from the bounds of hardcoded software, automate common analyses, and take advantages of the flow of data between functions. Pretty good for something that is entirely free!
This article is the first in a series on reliability that will explain key concepts and provide you with the code to repeat the analyses. This series isn’t designed to teach you Python, or to be a “how to” guide for using reliability (see the online documentation for that). Rather, it is to use reliability as tool to perform analyses that you may not have seen before due to the difficulty in conducting such analyses using GUI based software tools.
Great article. thanks for sharing